Tackling the Top 3 Challenging Programming Interview Questions
Written on
Chapter 1: Introduction
Recently, I stumbled upon a discussion highlighting the three most challenging questions someone faced during programming interviews. This motivated me to share my own experiences with difficult questions I encountered. For clarity, I will focus solely on the questions and omit detailed solutions.
It's essential to understand that solving programming challenges in front of an interviewer is often different from tackling them alone. Certain problems that seem straightforward can become much more complex under interview pressure. The main reason for this is the stress and anxiety that can arise when facing an interviewer. Thus, maintaining composure is crucial and can significantly impact your performance.
Here are three particularly challenging questions that tested my skills during interviews:
Section 1.1: Question 1
The first question was a standard one, yet I struggled with it due to the high-pressure environment of the interview. The problem was as follows:
You have a rectangular cake with dimensions x and y. Your task is to cut it into squares. In each move, you may select a rectangle and split it into two rectangles, ensuring all sides remain integers. What is the minimum number of moves required?
I encountered a similar question online, which helped me frame my understanding of the problem. Essentially, we are given a rectangle, and our goal is to cut it into smaller squares. For instance, consider a rectangle with dimensions 5 by 3.
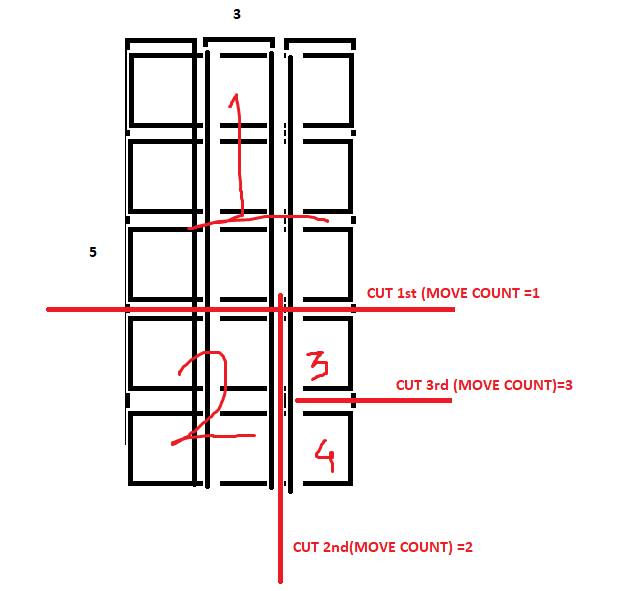
In this case, the rectangle can be divided into four smaller squares, requiring a minimum of three cuts. Thus, the expected output is three moves. This problem relates to dynamic programming, which should be approached efficiently and optimally.
Section 1.2: Question 2
The second question I faced came during a time when my programming experience was limited, making it particularly challenging. The problem was framed as follows:
Imagine you are a food delivery person tasked with transporting food from one location to another. You need to determine the shortest possible time while accounting for traffic lights at intersections. A red light adds an additional 5 seconds to your travel time, and adjacent traffic lights cannot both be red. The first light you encounter is red, and you are given a matrix that indicates the travel time between nodes.
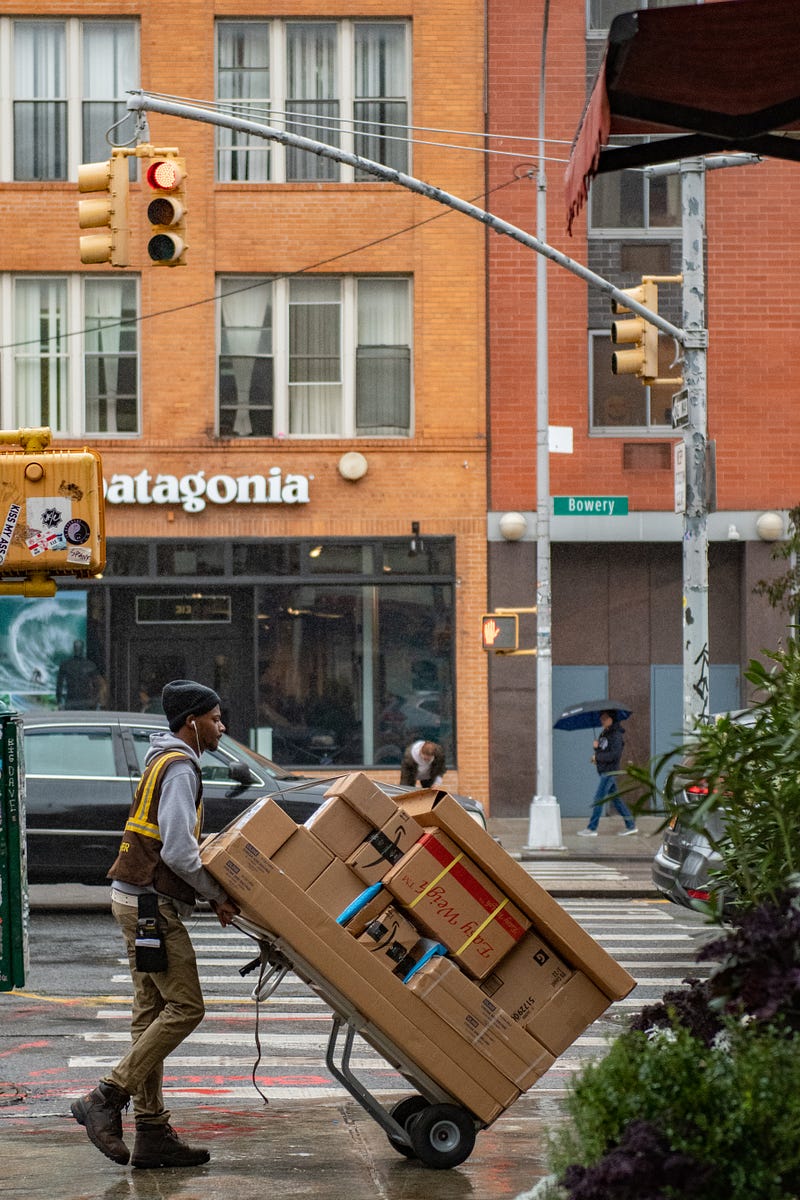
This question resembles a typical graph problem, where finding the optimal path is essential, and the complexity arises from the traffic light conditions. I found myself overwhelmed by the traffic light aspect during the interview. My advice is to remain calm and not overthink the situation.
Section 1.3: Question 3
The third question was quite intricate and posed a significant challenge regarding time complexity. Although I can't recall the exact wording, I was fortunate enough to find a similar problem online. The question was:
You are given an array of size n, and your task is to create x subarrays such that the maximum sum of any subarray is minimized. Essentially, you want to form x subarrays from the given array and report the smallest maximum sum.
The solution can be approached by processing the elements using binary search in conjunction with a greedy algorithm to determine the sum.
Conclusion
I hope you found these questions intriguing. With the right preparation and a calm mindset, you'll be better equipped to handle interviews successfully. Keep coding and practicing, and I wish you the best on your programming journey!
To enhance your knowledge in programming and technology, consider subscribing to my newsletter.
Watch this video titled "Ex-Google Software Engineer Speeds Through 3 Coding Interview Problems" to gain insights into tackling coding challenges effectively.
Check out this video, "Complete (250 questions) DSA Sheet for Coding Interviews | Leetcode questions," for a comprehensive review of coding interview questions.