How to Determine if a Value is NaN in JavaScript
Written on
Chapter 1: Understanding NaN in JavaScript
In JavaScript, there are occasions when we need to verify if a value is NaN (Not a Number). This article explores different methods to check for NaN values in JavaScript.
The following excerpt illustrates how to effectively check for NaN in JavaScript using several approaches.
Section 1.1: The isNaN Function
A common way to determine if a value is NaN is by utilizing the isNaN function. For example, we could write:
const nan = isNaN(parseFloat("abc"));
console.log(nan);
In this case, isNaN attempts to convert the argument into a number automatically. However, it's prudent to use parseFloat first for clarity. Since we provided a non-numeric string, parseFloat should yield NaN, resulting in isNaN returning true.
Subsection 1.1.1: Code Example
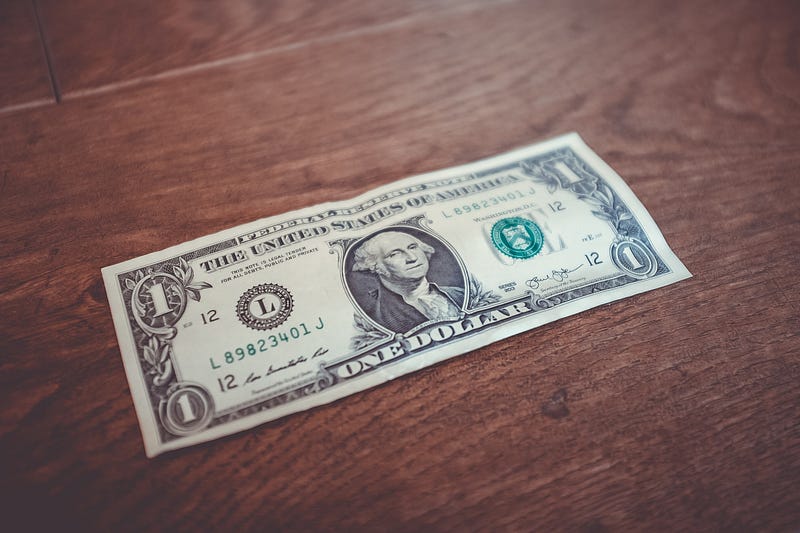
Section 1.2: Checking Self-Equality
In JavaScript, NaN is unique in that it does not equal itself. Therefore, we can verify whether a variable equals itself to determine if it holds a NaN value. For instance:
const nan = NaN;
console.log(nan !== nan);
The console will output true, confirming that NaN does not equal itself. If the variable holds any other value, the output would be false. For example:
const nan = true;
console.log(nan !== nan);
This would result in false.
Chapter 2: Alternative Methods to Check for NaN
The Number.isNaN Method
Another way to check if a value is NaN is through the Number.isNaN method. Unlike isNaN, this method does not attempt to convert the value into a number before checking. For example:
const nan = Number.isNaN('abc');
console.log(nan);
In this case, the output will be false because we did not provide NaN but rather a non-numeric string. Conversely:
const nan = Number.isNaN(NaN);
console.log(nan);
This will yield true.
The Object.is Method
Additionally, the Object.is method can be employed to check for NaN. It accepts two arguments to compare. If both are NaN, it returns true, unlike the strict equality operator (===), which does not recognize NaN as equal to itself. For example:
const a = NaN;
const nan = Object.is(a, NaN);
console.log(nan);
Here, the output will be true.
Conclusion
JavaScript provides various functions and methods to check for NaN. One effective approach is to utilize the strict equality operator (===) to see if a value is not equal to itself. If that condition holds, the value is confirmed as NaN, which is the only value in JavaScript that exhibits this behavior.